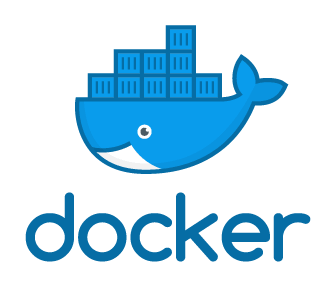
How do I connect to Postgresql running on host from Docker container
There could be instances where you want to connect to Postgresql database on the host from your containers. In this post, we take look at configuration steps on how to connect to Postgresql running on host from your Docker containers.
We are going to use Docker Compose tool to define and run multi-container applications. We would be defining compose configuration as YAML file for our application’s services/networks/volumes etc., and then with a single command, we can create and start all the services from the configuration.
Here are the key steps :
- DefineÂ
Dockerfile
 for your app’s environment. - DefineÂ
docker-compose.yml
 for the services that make up your app services. - Configure Postgresql to able to connect from Docker containers.
- RunÂ
docker-compose up
 and Compose starts and runs your entire app.
This quickstart assumes basic understanding of Docker concepts, please refer to earlier posts for understanding on Docker & how to install and containerize applications.
In the next section, we can look at how to define services in compose file for sample application with Angular as front end, Spring Boot as API, and for database as Postgres but this will be located on the host.
Quick Snapshot
#1.Definition of Docker files
We have already defined below docker files in the previous posts here &Â here. Create a new project directory and copy all dockerfiles to this folder.
Angular Application Docker file
FROM httpd:2.4 #copy angular dist folder to container COPY dist/ /usr/local/apache2/htdocs/ #copy htaccess and httpd.conf to container COPY .htaccess /usr/local/apache2/htdocs/ COPY httpd.conf /usr/local/apache2/conf/httpd.conf #change permissions RUN chmod -R 755 /usr/local/apache2/htdocs/ #expose port EXPOSE 4200
Spring Boot API Docker file
FROM openjdk:8-jre WORKDIR / #add required jars ADD spring-boot-rest-postgresql-0.0.1-SNAPSHOT.jar spring-boot-rest-postgresql-0.0.1-SNAPSHOT.jar #expose port EXPOSE 8080 #cmd to execute ENTRYPOINT ["java","-Djava.security.egd=file:/dev/./urandom","-jar","/spring-boot-rest-postgresql-0.0.1-SNAPSHOT.jar"]
#2.Define services in Docker compose file
Create a file called docker-compose.yml
 in your project directory and paste the following:
version: '3' services: ui: build: context: . dockerfile: UIDockerfile ports: - '4200:4200' networks: - samplenet links: - 'api:api' api: build: context: . dockerfile: AppDockerfile ports: - '8080:8080' networks: - samplenet networks: samplenet: null
Above compose file defines 2 services as below:
ui
: This is for angular application,it uses an image that’s built from the UIDockerfile in the current directory and forwards the exposed port4200
on the container to port4200
on the host machine.api
: This is for spring boot application, it uses an image that’s built from the AppDockerfile in the current directory and forwards the exposed port8080
on the container to port8080
on the host machine.- All the above services uses
samplenet
network depends_on
denotes the service dependencies. When you start the services, compose would start the dependent services as well.- If you have noticed, we haven’t created service for Postgresql application instead we would be using Postgresql database installed on the host.
- Also on your SpringBoot configuration (application.properties file) specify the external IP address of the database host likeÂ
spring.datasource.url=jdbc:postgresql://<IP Address>:5432/postgres
#3.Configure Postgresql to able to connect from Docker containers
Assuming Postgresql is already installed on the host machine, follow the below steps to configure the listen_addresses
on postgresql.conf
to accept all connections.

Also edit pg_hba.conf
 file to add entry for the host connections like below
host    all     all     0.0.0.0/0      md5

#4.Build and run your app with Compose
Once you have completed the above configurations,next step is to start the application.In the current project directory, run docker-compose up
.Compose pulls all the required Docker image, builds an image for your code, and starts the services you defined.


Congrats! today we have learned how to configure Postgresql running on the host to be able to connect from Docker containers.
Like this post? Don’t forget to share it!
Additional Resources:
- Official documentation as a reference to understand any command.
- Docker build reference
- Docker run reference
- Best Practices article on writing Docker files.
- Test your knowledge on Dockerfile.
- Full list of Compose commands
- Compose configuration file reference
- Take a free course on Building Scalable Java Microservices with Spring Boot and Spring Cloud
- Kubernetes tutorial – Scale & perform updates to your app
- Kubernetes tutorial – Create deployments using YAML file


Average Rating